Automation is the key to maximizing efficiency. If you’re managing a system, then there’s a good chance you’re performing several repetitive tasks daily. Bash scripting can help you automate these tasks and save significant time. Here, we’ll delve into ten real-world examples of how Bash scripts can assist in automating common system-related chores.
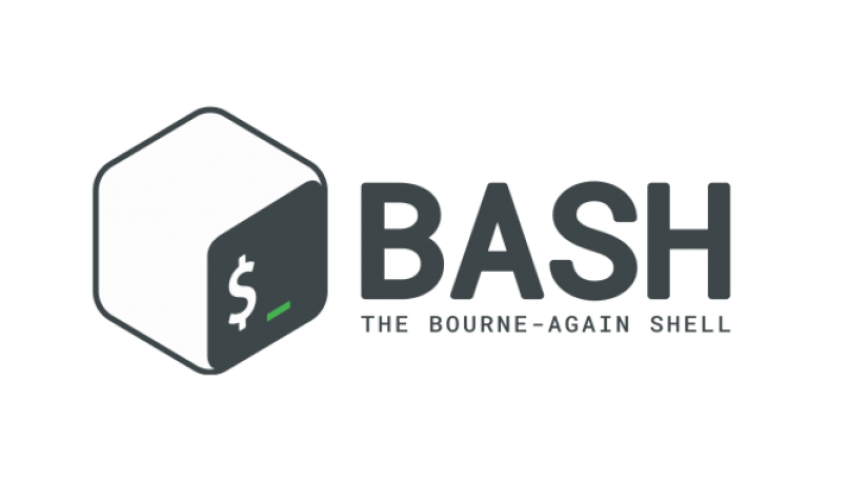
1. Disk Space Cleanup
Over time, systems can get cluttered with temporary and unnecessary files. Automating cleanup can free up space without manual intervention.
#!/bin/bash
# Remove all .tmp files from /tmp directory
find /tmp -type f -name '*.tmp' -delete
# Clear user cache
rm -rf ~/.cache/*
2. System Backup
A simple script can backup essential directories to an external drive or a remote server.
#!/bin/bash
tar czf /path/to/backup_dir/backup_$(date +%Y%m%d).tar.gz /path/to/important_dir/
3. Log Monitoring
Alert when a log file contains a specific string, indicating an error or important event.
#!/bin/bash
if grep -qi "error" /path/to/logfile.log; then
mail -s "Error Found" [email protected] < /path/to/logfile.log
fi
4. Update and Upgrade System
Keep your system updated without manual checks.
#!/bin/bash<br>sudo apt update && sudo apt upgrade -y
5. Database Backup
Automatically backup databases to ensure data integrity.
#!/bin/bash
mysqldump -u [username] -p[password] [database_name] > /path/to/backup/db_backup_$(date +%Y%m%d).sql
6. System Uptime Report
Check how long the system has been running and log it for records.
#!/bin/bash
uptime >> /path/to/uptime_log.txt
7. Automated File Sync
Sync a directory with a remote one using rsync
.
#!/bin/bash
rsync -avz /local/dir/ user@remote_server:/remote/dir/
8. Disk Usage Report
Get a report on directories consuming the most disk space.
#!/bin/bash
du -sh /* | sort -rh > /path/to/disk_usage_report.txt
9. Batch Image Resize
If you handle a lot of images, resizing can be cumbersome. Automate this using ImageMagick.
#!/bin/bash
mkdir resized_images
mogrify -path resized_images/ -resize 800x600 *.jpg
10. Daily Weather Report
Fetch and log daily weather information.
#!/bin/bash
curl wttr.in/YourCity?format=3 >> /path/to/weather_log.txt
When creating Bash scripts for automation:
- Always Test: Before letting a script run autonomously, always test it to ensure it behaves as expected.
- Use Cron:
cron
is a task scheduler in Unix-like systems. It’s perfect for running scripts at specified intervals. - Keep Learning: The examples given are basic. The more you dive into Bash scripting, the more advanced and useful scripts you can create.
Automating tasks not only saves time but also ensures that they are performed consistently. By investing a little time upfront in scripting, you can reap long-term benefits. Whether you’re a system admin, developer, or just someone looking to improve their workflow, Bash scripting is a powerful tool to have in your toolkit.
Leave a Reply