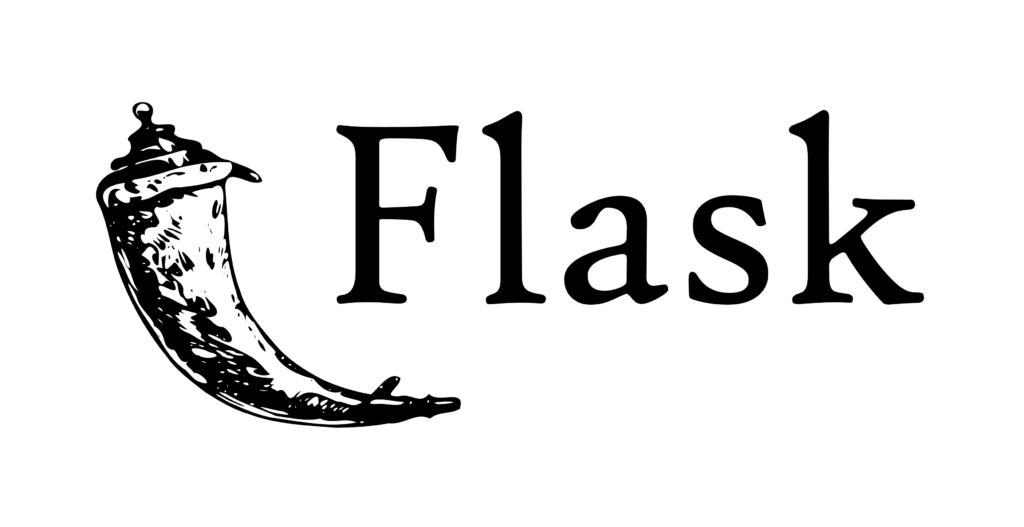
Introduction
Flask is a micro web framework written in Python. It’s lightweight, flexible, and perfect for building RESTful APIs quickly. In this guide, we’ll create a simple API with best practices on structuring and securing it.
Prerequisites
- Python (preferably 3.6+)
- pip (Python package installer)
1. Setting Up Your Environment
First, let’s set up a virtual environment for our project to keep our dependencies clean.
python3 -m venv myapp
source myapp/bin/activate
2. Installing Necessary Packages
Next, install Flask and Flask-RESTful which simplifies the creation of APIs.
pip install Flask Flask-RESTful
3. Structuring Your Application
For maintainability and scalability, we’ll structure our application in the following way:
/myapp
/app
__init__.py
/models
__init__.py
model.py
/resources
__init__.py
resource.py
run.py
4. Creating the Application
a) Initialize the Flask App (/app/__init__.py
):
from flask import Flask
from flask_restful import Api
app = Flask(__name__)
api = Api(app)
from app import resources
b) Define a Model (/app/models/model.py
):
While we won’t use a real database in this example, consider this as a placeholder for your data model.
class Model:
DATA = {
'myitem1': {'property': 'value1'},
'myitem2': {'property': 'value2'}
}
c) Create a Resource (/app/resources/resource.py
):
Resources define your endpoints.
from flask_restful import Resource
from app.models.model import Model
class Resource(Resource):
def get(self, item_name):
return {item_name: Model.DATA.get(item_name)}
# Add the route
from app import api
api.add_resource(Resource, '/item/<string:item_name>')
d) Run the Application (run.py
):
from app import app
if __name__ == '__main__':
app.run(debug=True)
5. Securing Your API
a) Using HTTPS:
Always use HTTPS for APIs, especially if sensitive data is being transferred. Consider using extensions like Flask-Talisman
for enforcing HTTPS.
b) API Authentication:
Flask provides several methods for authentication, like HTTP Basic Authentication or token-based authentication. For more advanced authentication, you can use extensions like Flask-JWT
or Flask-Login
.
c) Rate Limiting:
Protect your API from abuse by adding rate limits. You can use the Flask-Limiter
extension for this purpose.
d) Error Handling:
Always handle errors gracefully. Don’t expose stack traces to the client. Instead, return a user-friendly error message.
e) CORS Handling:
If your API will be accessed from different origins, consider using the Flask-CORS
extension to handle Cross-Origin Resource Sharing (CORS) settings.
6. Testing Your API
With everything set up, you can run your Flask application:
python run.py
Visit http://127.0.0.1:5000/item/item1
in your browser or use tools like curl
or Postman to make a GET request. You should receive a JSON response.
Conclusion
This is a basic introduction to creating RESTful APIs with Flask. As you delve deeper into building APIs, you might want to look into more advanced topics such as using ORM (like SQLAlchemy), pagination, caching, and logging for better performance, scalability, and maintainability.
Leave a Reply